react-native-flip-timer
A Flip timer implementation in React Native.
Show Cases
IOS |
Android |
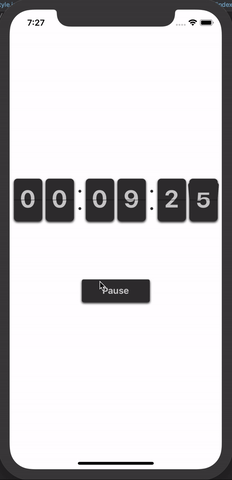 |
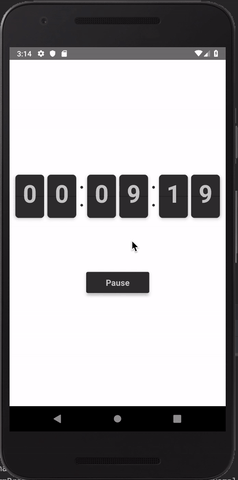 |
Installation
$ npm i react-native-flip-timer --save
Basic Usage
import React, { Component } from 'react';
import {
StyleSheet, View, TouchableOpacity, Text,
} from 'react-native';
import { Timer, FlipNumber } from 'react-native-flip-timer';
export default class App extends Component {
state = {
play: true,
}
play = () => {
this.setState(({ play }) => ({ play: !play }));
}
render() {
const { play } = this.state;
return (
<View style={styles.container}>
<Timer time={500} play={play} />
<TouchableOpacity style={styles.button} onPress={this.play}>
<Text style={styles.text}>{play ? 'Pause' : 'Play'}</Text>
</TouchableOpacity>
</View>
);
}
}
const styles = StyleSheet.create({
container: {
flex: 1,
backgroundColor: 'white',
alignItems: 'center',
justifyContent: 'center',
},
button: {
height: 40,
backgroundColor: '#333333',
width: 120,
alignItems: 'center',
justifyContent: 'center',
},
text: {
fontSize: 16,
fontWeight: 'bold',
color: '#cccccc',
},
});
Properties
Timer Props
Prop |
Default |
Type |
Description |
time |
required |
string / number |
Time (in seconds) |
play |
true |
bool |
Play the timer |
wrapperStyle |
{} |
object |
Wrapper for the Timer |
Flip Number Props
Prop |
Default |
Type |
Description |
number |
required |
string / number |
Number Input |
unit |
seconds |
hours / minutes / seconds |
Number Input Unit |
perspective |
250 |
number |
Perspective |
numberWrapperStyle |
{} |
object |
Wrapper Style |
cardStyle |
{} |
object |
Card Style |
flipCardStyle |
{} |
object |
Flip Card Style |
numberStyle |
{} |
object |
Number Style |
Todos
- Full Coverage Tests for the Components
- Support for Labels
GitHub