React Native Images Collage
Pure JavaScript image collage component for React Native.
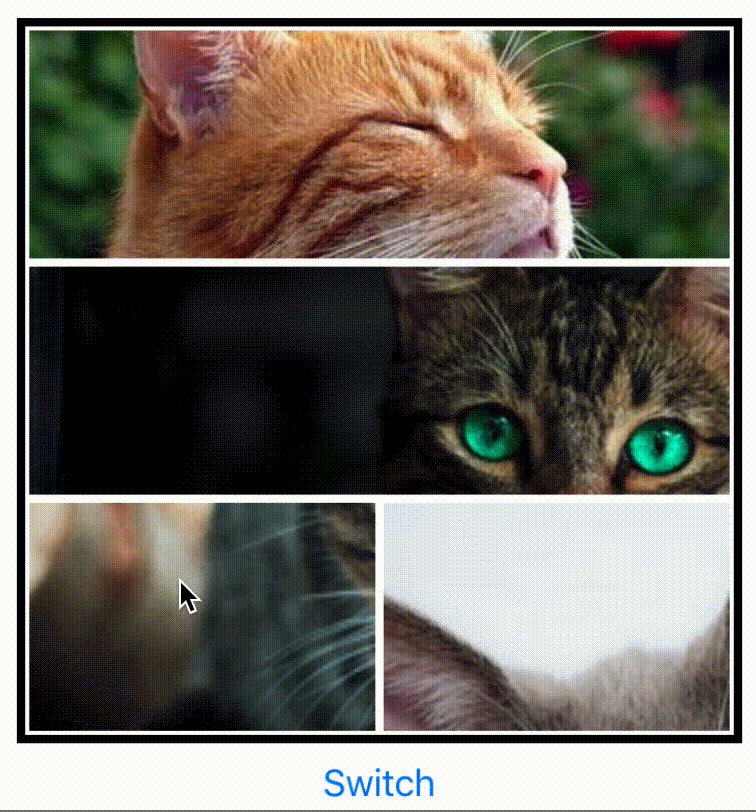



Install
To get started install via npm:
npm install react-native-images-collage --save
Usage
Dynamic and Static Collage
To use in React Native. Import:
import { DynamicCollage, StaticCollage } from './react-native-images-collage';
Then add this to your code:
<DynamicCollage
width={Dimensions.get('window').width / 1.1}
height={Dimensions.get('window').width / 1.1}
images={ photos }
matrix={ [ 1, 1, 1, 1 ] } />
StaticCollage does not include any panning, scaling or arrangement logic. Used this is if you want to render multiple non-interactive collages. StaticCollage takes the same props as DynamicCollage.
Layouts
Instead of building your own matrix of collage layouts. There is a JSON file you can import which includes multiple layouts, up to 6 images.
import { LayoutData } from 'react-native-images-collage';
You can then access a layout like so:
matrix={ LayoutData[NumberOfImages][i].matrix }
direction={ LayoutData[NumberOfImages][i].direction }
The number in the first bracket will be the configuration you want to access. E.g. configuration for 5 images. The second number is the specific layout you want to access e.g. [2, 2, 1]. You will have to inspect the JSON file to find this out.
Notes
If you want to capture the collage as a single image. Take a look at react-native-view-shot.
Props
Note: For this to work as expected, the number of images has to be equal to the result of all numbers in the matrix. e.g. if matrix is [ 1, 2, 1 ] ( 1 + 2 + 1 = 4), there has to be 4 images.
Prop | Type | Optional | Default | Description |
---|---|---|---|---|
width | float | No | Width of component. Not optional. Used to calculate image boundaries for switching | |
height | float | No | Height of component. Not optional. Used to calculate image boundaries for switching | |
images | array | No | Images for the collage. | |
matrix | centered | No | An array [ 1, 1, 1 ] equal to the number of images. Used to define the layout | |
separators | int | Yes | 0 | Amount of space between images. |
direction | string | Yes | row | Direction of the collage: 'row' or 'column'. |
borders | int | Yes | 4 | Width of borders. |
borderColor | string | Yes | white | Border colour. |
backgroundColor | string | Yes | white | Background color of collage. |
containerStyle | object | Optional | 100% | Style applied to the container of the collage |
Todo
- [ ] Rewrite, so it works better.
- [ ] Add to awesome-react-native
- [ ] Need to work on performance. Reducing set state and optimizing code.
- [ ] Fix various scaling issues
- [ ] Test on Android