react-native-qrcode-svg
A QR Code generator for React Native based on react-native-svg and javascript-qrcode.
Features
- Easily render QR code images
- Optionally embed a logotype
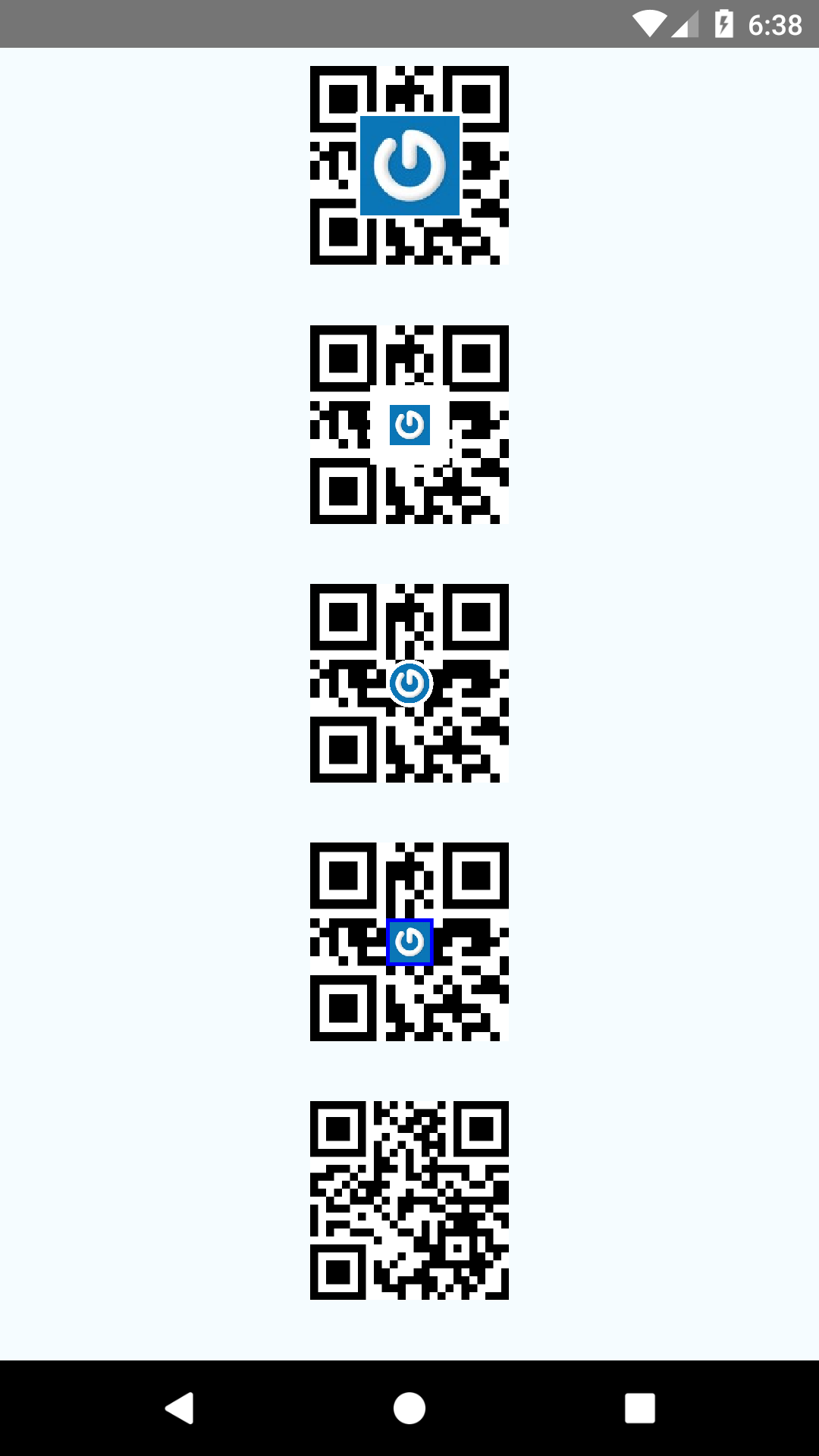
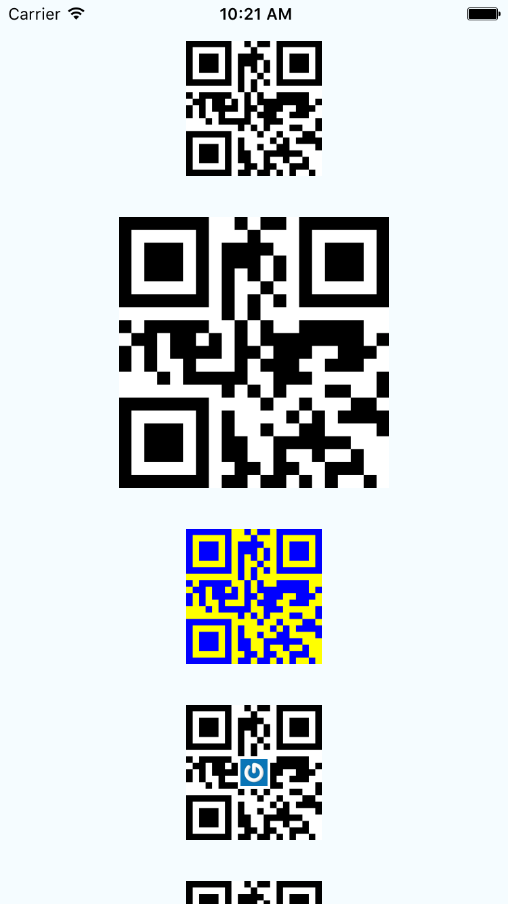

Installation
Please install react-native-svg first.
npm install react-native-svg --save
react-native link react-native-svg
npm install react-native-qrcode-svg --save
Examples
import QRCode from 'react-native-qrcode-svg';
//Simple usage, defaults for all but the value
render() {
return (
<QRCode
value="http://awesome.link.qr"
/>
);
};
// 30px logo from base64 string with transparent background
render() {
let base64Logo = 'data:image/png;base64,iVBORw0KGgoAAAANSUhEUgAAAOEAA..';
return (
<QRCode
value="Just some string value"
logo={{uri: base64Logo}
logoSize={30}
logoBackgroundColor='transparent'
/>
);
};
// 20% (default) sized logo from local file string with white logo backdrop
render() {
let logoFromFile = require('../assets/logo.png');
return (
<QRCode
value="Just some string value"
logo={logoFromFile}
/>
);
};
// get base64 string encode of the qrcode (currently logo is not included)
getDataURL() {
this.svg.toDataURL(this.callback);
}
callback(dataURL) {
console.log(dataURL);
}
render() {
return (
<QRCode
value="Just some string value"
getRef={(c) => (this.svg = c)}
/>
);
}
Props
Name | Default | Description |
---|---|---|
size | 100 | Size of rendered image in pixels |
value | 'this is a QR code' | Value of the QR code |
color | 'black' | Color of the QR code |
logo | null | Image source object. Ex. {uri: 'base64string'} or {require('pathToImage')} |
logoSize | 20% of size | Size of the imprinted logo. Bigger logo = less error correction in QR code |
logoBackgroundColor | backgroundColor | The logo gets a filled quadratic background with this color. Use 'transparent' if your logo already has its own backdrop. |
logoMargin | 2 | logo's distance to its wrapper |
logoBorderRadius | null | the border-radius of logo image (Android is not supported) |
getRef | null | Get SVG ref for further usage |
ecl | 'M' | Error correction level |
Saving generated code to gallery
Note: Experimental only ( not tested on iOS) , uses getRef() and needs RNFS module
npm install --save react-native-fs
Example for Android:
import { CameraRoll , ToastAndroid } from "react-native"
import RNFS from "react-native-fs"
...
saveQrToDisk() {
this.svg.toDataURL((data) => {
RNFS.writeFile(RNFS.CachesDirectoryPath+"/some-name.png", data, 'base64')
.then((success) => {
return CameraRoll.saveToCameraRoll(RNFS.CachesDirectoryPath+"/some-name.png", 'photo')
})
.then(() => {
this.setState({ busy: false, imageSaved: true })
ToastAndroid.show('Saved to gallery !!', ToastAndroid.SHORT)
})
})
}